728x90
반응형
[ 연습문제 ]
Practice 6
1번: 구구단 2단 만들기
- 반복문을 활용하여 구구단 2단 출력
답안
더보기
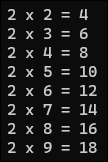
for (int i = 2; i <= 9; i++)
{
Console.WriteLine("2 x "+i+" = "+ i * 2);
}
결과
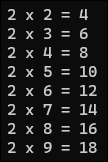
2번: 입력받은 데이터로 구구단 만들기
- 프로그램 실행 시 출력하고 싶은 단을 입력해 주세요. 메시지 출력
- 하나의 수 입력 후 엔터
- 입력받은 데이터가 숫자라면 2~9를 곱한 결과 출력
- 입력받은 데이터가 숫자가 아니라면 숫자가 아닙니다. 메시지 출력
답안
더보기
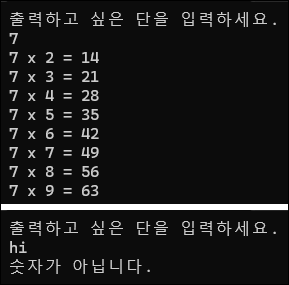
Console.WriteLine("출력하고 싶은 단을 입력하세요.");
string input = Console.ReadLine();
int num;
bool isInt = int.TryParse(input, out num);
if (isInt)
{
for (int i = 2; i <= 9; i++)
{
Console.WriteLine(num + " x " + i + " = " + num * i);
}
}
else
{
Console.WriteLine("숫자가 아닙니다.");
}
결과
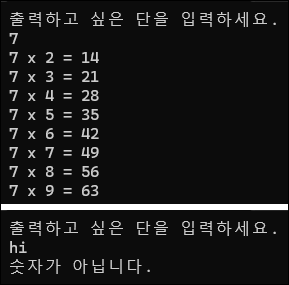
3번: 피보나치 수열 구하기
- Console.Write();를 활용하여 피보나치 수열 10개까지 출력
- Console.Write();은 Console.WriteLine();과 다르게 줄을 바꾸지 않고 옆으로 출력한다.
답안
더보기

int first = 0;
int second = 1;
int third;
int count = 0;
while(count < 10)
{
Console.Write(second+" ");
third = first + second;
first = second;
second = third;
count++;
}
결과

4번: 입력받은 수만큼 피보나치 수열 구하기
- 프로그램 실행 시 몇 개의 피보나치 수열을 출력하고 싶으신가요? 메시지 출력
- 하나의 수 입력 후 엔터
- 입력받은 데이터가 숫자라면
- 숫자가 0 이하라면 양수를 입력해 주세요. 메시지 출력
- 숫자가 47 이상이라면 숫자가 너무 큽니다. 메시지 출력
※피보나치 수열의 47번째 수부터는 int의 범위를 벗어남
- 입력받은 데이터가 숫자가 아니라면 숫자가 아닙니다. 메시지 출력
답안
더보기

Console.WriteLine("몇개의 피보나치 수열을 출력하고 싶으신가요?");
string input = Console.ReadLine();
int i;
bool isInt = int.TryParse(input, out i);
if (isInt)
{
if (i <= 0)
{
Console.WriteLine("양수를 입력해 주세요.");
}
else if (i >= 47)
{
Console.WriteLine("숫자가 너무 큽니다.");
}
else
{
int first = 0;
int second = 1;
int count = 0;
while (count < i)
{
Console.Write(second + " ");
int result = first + second;
first = second;
second = result;
count++;
}
}
}
else
{
Console.WriteLine("숫자가 아닙니다.");
}
결과

Practice 7
1번: 3글자 이상 10글자 이하의 이름을 입력할 수 있는 프로그램 작성
- 프로그램 실행 시 이름을 입력해 주세요. (3~10글자) 메시지 출력
- 이름이 3글자 미만, 10글자 초과라면 이름을 확해 주세요. 메시지 출력
- 올바르게 입력했다면 안녕하세요! 제 이름은 입력받은 데이터입니다. 메시지 출력
- ___.Length를 활용하면 문자열의 길이를 알 수 있다. 밑줄에는 문자열 변수명을 넣는다.
답안
더보기
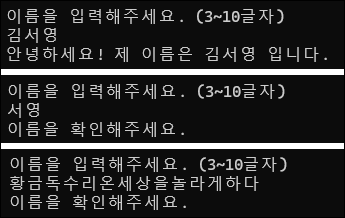
Console.WriteLine("이름을 입력해주세요. (3~10글자)");
string input = Console.ReadLine();
int length = input.Length;
if (length >= 3 && length <=10)
{
Console.WriteLine("안녕하세요! 제 이름은 " + input + " 입니다.");
}
else
{
Console.WriteLine("이름을 확인해주세요.");
}
결과
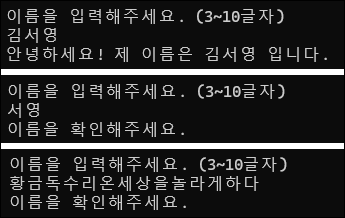
2번: 조건에 맞을 때까지 이름 입력
- 1번의 프로그램에서 3~10글자의 이름을 입력하지 않았을 때 프로그램이 종료되지 않고, 올바르게 입력할 때까지 반복되게 실행
- 반복문과 bool을 활용
답안
더보기
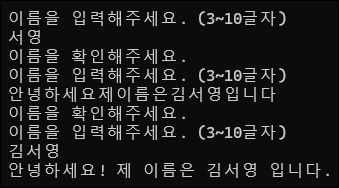
bool name;
do
{
Console.WriteLine("이름을 입력해주세요. (3~10글자)");
string input = Console.ReadLine();
int length = input.Length;
name = length >= 3 && length <= 10;
if (name)
{
Console.WriteLine("안녕하세요! 제 이름은 " + input + " 입니다.");
}
else
{
Console.WriteLine("이름을 확인해주세요.");
}
}
while (!name);
결과
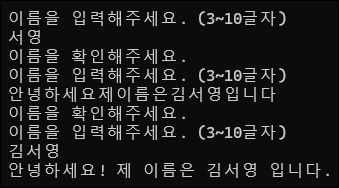
3번: 반복 시 기존 내용 지우기
- Console.Clear();를 활용하여 프로그램을 계속해서 실행했을 때, 콘솔에 보여지는 반복된 문장이 지워지게 실행
- Console.Clear();는 콘솔에 표시된 내용들을 모두 지운다.
답안
더보기
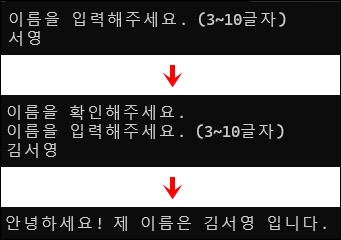
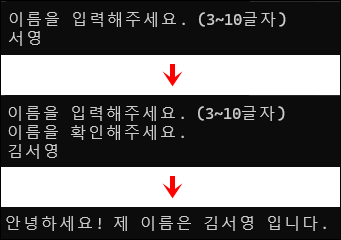
bool name;
do
{
Console.WriteLine("이름을 입력해주세요. (3~10글자)");
string input = Console.ReadLine();
int length = input.Length;
name = length >= 3 && length <= 10;
Console.Clear();
if (name)
{
Console.WriteLine("안녕하세요! 제 이름은 " + input + " 입니다.");
}
else
{
Console.WriteLine("이름을 확인해주세요.");
}
}
while (!name);
결과
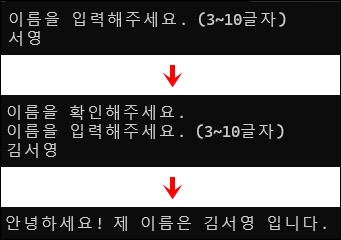
- 처음에 출력되는 이름을 입력해주세요. (3~10글자) 문장이 계속 위로 가게 수정한 코드
bool name;
string sentence = "이름을 입력해주세요. (3~10글자)";
Console.WriteLine(sentence);
do
{
string input = Console.ReadLine();
int length = input.Length;
name = length >= 3 && length <= 10;
Console.Clear();
Console.WriteLine(sentence);
if (name)
{
Console.Clear();
Console.WriteLine("안녕하세요! 제 이름은 " + input + " 입니다.");
}
else
{
Console.WriteLine("이름을 확인해주세요.");
}
}
while (!name);
결과
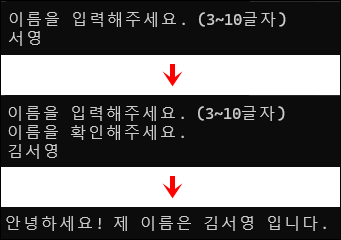
728x90
반응형
'Coding > C#' 카테고리의 다른 글
[내일배움캠프 6일차 TIL] C#, 객체 지향, 변수, 자료형, 형변환 (0) | 2024.04.22 |
---|---|
C# 배열 생성 및 데이터 저장, 접근, 배열 리터럴, 인덱스 (0) | 2024.04.13 |
C# 반복문 for, while, do while, break, continue (0) | 2024.04.13 |
C# 조건문 연습 문제 (0) | 2024.04.13 |
C# 조건문 if, else if, else, switch case, 조건부 논리 연산자 (0) | 2024.04.03 |